Arduino DHT22 readings over serial
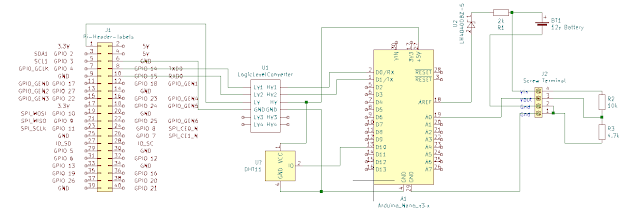
Today I am going to add a DHT22 temperature humidity sensor to my existing battery voltage monitor project. Uploading and testing the code is done over USB cable, the HC-05 needs to be disconnected for this to work. The DHT22 is connected to +5v, gnd and Digital Pin 10, I only chose pin10 as it is convenient for my veroboard layout. The code for this is very simple : <code> #include "DHT.h" #define DHTPIN 2 // The pin that your DHT is connected to DHT dht(DHTPIN, DHT22); void setup() { dht.begin(); } void loop(){ float h = dht.readHumidity(); //stores humidity value in float variable h float t = dht.readTemperature();// temperature value } </code> Don't forget to import the "DHT sensor library" in library manager in the Arduino IDE. We can then do whatever we need to with the values. I am going to send request them over serial via a HC-05 Bluetooth device from a Python script on a Raspberry Pi. For testing am using a laptop running Linux Mint